The basic sequence of getting tokens is as follows:
- A client sends a username/password combination to the server.
- The server validates the authentication.
- If authentication is successful, the server creates a JWT token and refresh token else establishes an error response.
- On successful authentication, the client gets a JWT token in the response body and a refresh token in the cookie with all cookie protection flags like (httpOnly, secure=true, and SameSite=strict flag [whenever possible to prevent CSRF]).
- The client stores the access token in-memory. It means that you put this access token in a variable in your front-end site (like const accessToken = xyz). Yes, this means that the access token will be gone if the user switches tabs or refreshes the site. That’s why we have the refresh token. We’re not putting this access token in localStorage or cookie via JavaScript because it’s easier for attackers to dump that data, making it more prone to be stolen via an XSS attack. We can also use the access token in cookies with all secure flags, but the problem is that the access token is mostly big in size and the cookie size limit is 4kb. Check this blog.
- From next time, the client for making any request supplies the JWT token in request headers like this. Authorization: Bearer <jwt_token>
The server, upon receiving the JWT validates it and sends the successful response else error.
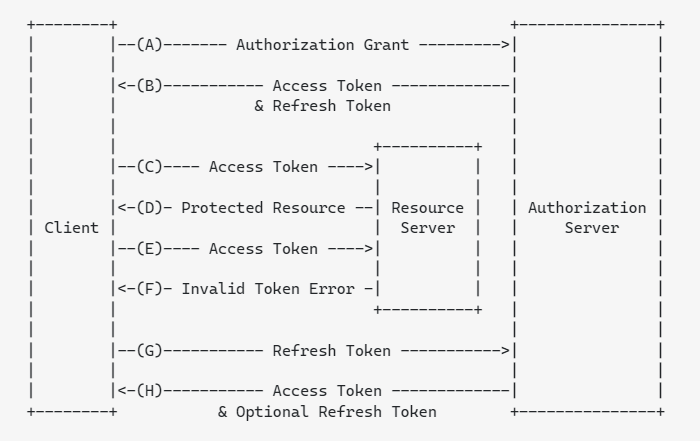
(A) The client requests an access token by authenticating with the authorization server.
(B) The authorization server authenticates the client and issues an access token and a refresh token.
(C ) The client makes a protected resource request to the resource server by presenting the access token.
(D) The resource server validates the access token, and if valid, serves the request.
(E) Steps (C )and (D) repeat until the access token expires. If the client knows the access token expired, it skips to step (G); otherwise, it makes another protected resource request.
(F) Since the access token is invalid, the resource server returns an invalid token error.
(G) The client requests a new access token by authenticating with the authorization server and presenting the refresh token. The client authentication requirements are based on the client type and on the authorization server policies.
(H) The authorization server authenticates the client and validates the refresh token, and if valid, issues a new access token (and, optionally, a new refresh token).
For more detail on JWT:
https://lazyhacker22.blogspot.com/2022/06/what-is-jwt-json-web-tokens-simple.html
https://lazyhacker22.blogspot.com/2022/06/what-is-jwt-json-web-tokens-simple.html
Comments
Post a Comment